fft
Fast Fourier Transform.
Syntax
f = fft(x)
f = fft(x,n)
f = fft(x,n,dim)
Inputs
- x
- The signal to be transformed into the frequency domain.
- n
- Size of the fft.
- dim
- The dimension on which to operate.
Outputs
- f
- The frequency domain representation of x.
Example
fft of signal with two frequency components.
The example shows how to interpret spectral vector locations. The two-sided spectrum is shown. The DC response is at 0, and 50 is the Nyquist frequency. The positive frequency responses are displayed in the range [5,50], and the negative frequency responses are displayed in the range [55,95] by convention.
f1 = 25; % first frequency component
f2 = 40; % second frequency component
fs = 100; % sampling frequency
ts = 1/fs; % sampling time interval
n = 20; % number of samples
time = [0:ts:(n-1)*ts]; % time vector
signal = sin(2*pi*f1*time) + 0.8 * sin(2*pi*f2*time);
fq = freq(n,fs); % frequency vector
ft = fft(signal) / n; % normalized fft
plot(fq, abs(ft));
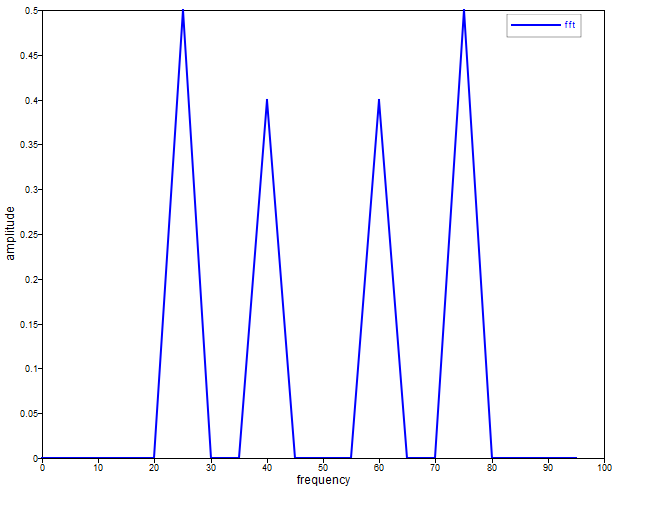
Figure 1. fft figure 1
The frequency spacing is 5 Hz, so both components fall exactly on one of the frequency vector values.
Comments
The fft is applied to a vector input, or to each column vector of a matrix input. The n frequencies associated with the outputs are spaced in increments of fs/n, where fs is the sampling frequency. The frequencies span from 0 to fs*[(n-1)/n]. A common use of fft is to prepare a time domain signal for the application of a frequency domain filter. The size of the fft, n, defaults to the length of the vector being transformed. If n is not the default, the signal vector is either truncated or extended with zeros to the specified length. The effect on the frequency spectrum is to insert additional samples symmetrically with respect to the Nyquist frequency. Raising the value of n beyond the default value effectively treats the original data with a rectangular window and extends the time span. The effect is that the original frequency spectrum is interpolated to determine output values with a denser spacing. This may be useful if the original frequency spacing is sparse so that significant frequency content falls between values in the frequency vector. fft uses the FFTW library.